Bypass ad blockers for Vercel Analytics in Next.js
If you're using Vercel Analytics with Next.js, chances are you're losing half of your analytics data. Why? Most modern browsers come with built-in ad blockers or tracking protection, which block requests from known analytics services, including Vercel Analytics.
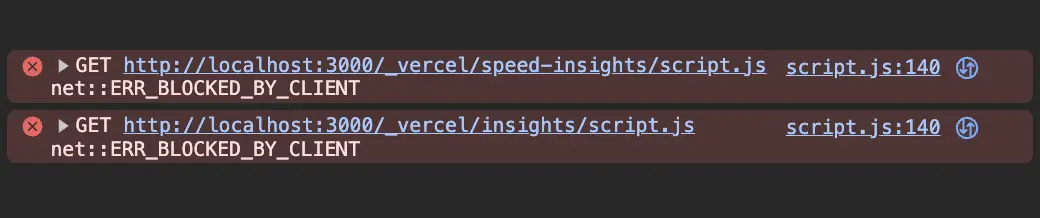
When you encounter ERR_BLOCKED_BY_CLIENT
errors in your console, it means an ad blocker is at work. In this case, it's blocking Vercel's Analytics and Speed Insights scripts.
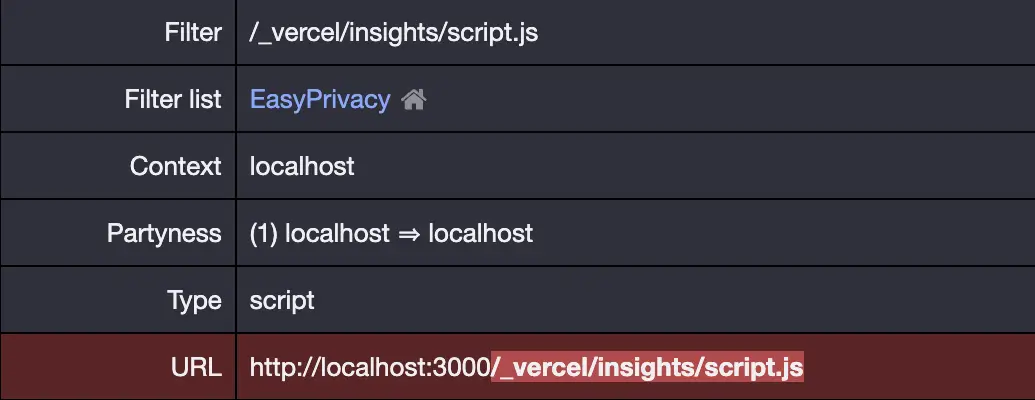
The request to /_vercel/insights/script.js
are blocked because it's in the filter list. Consequently, the script doesn't load, and analytics data isn't sent.
The Solution
You can prevent Vercel Analytics from being blocked by making the analytics request URLs less recognizable. One simple method is using Next.js config's rewrites.
Step 1: Create a Rewrite Rule
Rewrite all requests from /api/data/*
to /_vercel/insights/*
. Ensure the destination URL includes your domain name as that's where analytics requests go in production. The blocker will not notice requests to /api/data/*
, while Next.js will handle them and forward them to the actual analytics script.
Step 2: Create a Custom Analytics Script
Inject a custom script to send requests to our rewritten endpoint. Set the data-endpoint
attribute in the second script to the custom endpoint we've created in the rewrite rule.
This component loads the Vercel Analytics script from our custom endpoint /api/data/script.js
. In development mode, it loads the debug script from Vercel's CDN to test analytics locally without affecting production data.
This solution has not been tested with the custom events feature. You may need to adjust the rewrites and the script to work with custom events.
Step 3: Include the Custom Script in Your Layout
Step 4: Deploy and Test
Deploy your changes and test if analytics data is sent correctly. Check the network tab in your browser's developer tools for requests sent to the correct endpoint. Adjust the custom endpoint if requests are still blocked.
If you had @vercel/analytics
previously installed, you can safely uninstall
it as the script now loads from a custom endpoint.
Bonus Tip: Adding Vercel's Speed Insights
Enabling Vercel's Speed Insights with the same method is as simple as adding another rewrite rule and a custom script.
Add rewrite rules for Speed Insights:
Define a custom script to load the Speed Insights script from the custom endpoint. Make sure the data-endpoint
attribute ends with /vitals
to match the Speed Insights script's default endpoint.
And finally, include the custom script in your layout.
This method can be adapted for other frameworks or analytics services by rewriting requests to a custom endpoint and loading scripts from there.